| 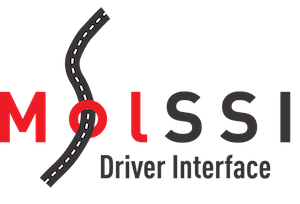 |
Molssi Driver Interface Library
|
Go to the documentation of this file.
33 int mpi_send_msg(
const void* buf,
int count, MDI_Datatype datatype, MDI_Comm comm);
34 int mpi_recv_msg(
void* buf,
int count, MDI_Datatype datatype, MDI_Comm comm);
35 int mpi_send(
const void* buf,
int count, MDI_Datatype datatype, MDI_Comm comm,
int msg_flag);
36 int mpi_recv(
void* buf,
int count, MDI_Datatype datatype, MDI_Comm comm,
int msg_flag);
int set_world_size(int world_size_in)
Set the size of MPI_COMM_WORLD.
Definition: mdi_mpi.c:21
int mpi_on_send_command(const char *command, MDI_Comm comm, int *skip_flag)
Callback when the MPI method must send a command.
Definition: mdi_mpi.c:213
int mpi_send(const void *buf, int count, MDI_Datatype datatype, MDI_Comm comm, int msg_flag)
Send data through an MDI connection, using MPI.
Definition: mdi_mpi.c:552
int mpi_rank
The rank of this process within the inter-code MPI communicator.
Definition: mdi_mpi.h:16
int communicator_delete_mpi(void *comm)
Function for MPI-specific deletion operations for communicator deletion.
Definition: mdi_mpi.c:650
int mpi_on_selection()
Callback when the end-user selects MPI as the method.
Definition: mdi_mpi.c:82
MPI_Comm mpi_comm
Inter-code MPI communicator.
Definition: mdi_mpi.h:14
int mpi_update_world_comm(void *world_comm)
Update a pointer to MPI_COMM_WORLD to instead point to the intra-code MPI communicator.
Definition: mdi_mpi.c:520
int mpi_after_send_command(const char *command, MDI_Comm comm)
Callback after the MPI method has received a command.
Definition: mdi_mpi.c:220
int mpi_on_accept_communicator()
Callback when the MPI method must accept a communicator.
Definition: mdi_mpi.c:183
int enable_mpi_support(int code_id)
Enable support for the TCP method.
Definition: mdi_mpi.c:58
int set_world_rank(int world_rank_in)
Set the rank of this process in MPI_COMM_WORLD.
Definition: mdi_mpi.c:41
int mpi_recv(void *buf, int count, MDI_Datatype datatype, MDI_Comm comm, int msg_flag)
Receive data through an MDI connection, using MPI.
Definition: mdi_mpi.c:608
int mpi_identify_codes(const char *code_name, int use_mpi4py, MPI_Comm world_comm)
Identify groups of processes belonging to the same codes.
Definition: mdi_mpi.c:268
int mpi_on_recv_command(MDI_Comm comm)
Callback when the MPI method must receive a command.
Definition: mdi_mpi.c:248
int use_mpi4py
Flag whether to use mpi4py instead of the linked MPI library.
Definition: mdi_mpi.h:18