| 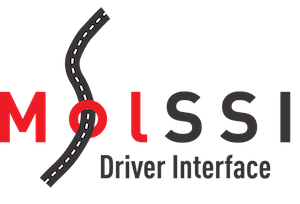 |
Molssi Driver Interface Library
|
Go to the documentation of this file.
19 #define mdi_strdup _strdup
21 #define mdi_strdup strdup
25 #define PLUGIN_PATH_LENGTH 2048
28 #define MDI_LANGUAGE_C 1
29 #define MDI_LANGUAGE_FORTRAN 2
30 #define MDI_LANGUAGE_PYTHON 3
33 #define MDI_MAJOR_VERSION_ 1
34 #define MDI_MINOR_VERSION_ 4
35 #define MDI_PATCH_VERSION_ 19
38 #define MDI_COMMAND_LENGTH_ 256
41 #define MDI_NAME_LENGTH_ 256
44 #define MDI_LABEL_LENGTH_ 64
47 #define MDI_COMM_NULL_ 0
52 #define MDI_INT16_T_ 8
53 #define MDI_INT32_T_ 9
54 #define MDI_INT64_T_ 10
55 #define MDI_UINT8_T_ 11
56 #define MDI_UINT16_T_ 12
57 #define MDI_UINT32_T_ 13
58 #define MDI_UINT64_T_ 14
75 typedef int MDI_Comm_Type;
76 typedef int MDI_Datatype_Type;
77 typedef int (*MDI_execute_command_type)(
void*, MDI_Comm_Type,
void*);
122 int (*on_accept_communicator)();
123 int (*on_send_command)(
const char*, MDI_Comm_Type,
int* skip_flag);
124 int (*after_send_command)(
const char*, MDI_Comm_Type);
125 int (*on_recv_command)(MDI_Comm_Type);
140 int (*
send)(
const void*, int, MDI_Datatype_Type, MDI_Comm_Type, int);
142 int (*
recv)(
void*, int, MDI_Datatype_Type, MDI_Comm_Type, int);
144 int (*
delete)(
void*);
163 char name[MDI_COMMAND_LENGTH_];
293 int new_method(
size_t code_id,
int method_id,
int* id_ptr);
309 int datatype_info(MDI_Datatype_Type datatype,
size_t* size, MDI_Datatype_Type* base);
316 void* sendbuf_in, MDI_Datatype_Type sendtype,
int get_node_index(vector *v, const char *node_name, int *node_index)
Determine the index of a node within a vector of nodes.
Definition: mdi_global.c:163
int communicator_delete(void *comm)
Dummy function for method-specific deletion operations for communicator deletion.
Definition: mdi_global.c:763
int * plugin_argc_ptr
Argument count for plugin command-line options.
Definition: mdi_global.h:186
int vector_free(vector *v)
Free all data associated with a vector.
Definition: mdi_global.c:127
size_t capacity
Total number of elements that can be stored by this vector.
Definition: mdi_global.h:93
char role[MDI_NAME_LENGTH_]
Role of the driver/engine.
Definition: mdi_global.h:174
vector * commands
Vector containing all the commands supported at this node.
Definition: mdi_global.h:165
int returned_comms
The number of communicator handles that have been returned by MDI_Accept_Connection()
Definition: mdi_global.h:237
Definition: mdi_global.h:132
sock_t sockfd
For communicators using the TCP communicatiom method, the socket descriptor (WINDOWS)
Definition: mdi_global.h:148
void * shared_state_from_driver
Shared plugin state, received from the driver during plugin calculations.
Definition: mdi_global.h:192
int(* mpi4py_size_callback)(int)
Python callback pointer for MPI_Comm_size.
Definition: mdi_global.h:216
int free_node_vector(vector *v)
Determine the index of a callback within a node.
Definition: mdi_global.c:239
void * method_data
Method-specific information for this communicator.
Definition: mdi_global.h:138
void mdi_error(const char *message)
Print error message.
Definition: mdi_global.c:799
char name[MDI_NAME_LENGTH_]
Name of the driver/engine.
Definition: mdi_global.h:172
int vector_get(vector *v, int index, void **element)
Return a pointer to an element of a vector.
Definition: mdi_global.c:147
int(* send)(const void *, int, MDI_Datatype_Type, MDI_Comm_Type, int)
Function pointer for method-specific send operations.
Definition: mdi_global.h:140
vector * comms
Vector containing all communicators associated with this code.
Definition: mdi_global.h:180
int(* recv)(void *, int, MDI_Datatype_Type, MDI_Comm_Type, int)
Function pointer for method-specific receive operations.
Definition: mdi_global.h:142
int(* mpi4py_allgather_callback)(void *, void *)
Python callback pointer for the initial MPI allgather.
Definition: mdi_global.h:208
int new_communicator(size_t code_id, int method, MDI_Comm_Type *comm_id_ptr)
Create a new communicator structure and add it to the list of communicators Returns the handle of the...
Definition: mdi_global.c:619
int initialized
Flag whether the vector has been initialized.
Definition: mdi_global.h:99
int mpi_initialized
Flag whether this code has previously initialized MPI.
Definition: mdi_global.h:255
int file_exists(const char *file_name, int *flag)
Check whether a file exists.
Definition: mdi_global.c:773
int(* mpi4py_split_callback)(int, int, MDI_Comm_Type, int)
Python callback pointer for MPI_Comm_split.
Definition: mdi_global.h:212
MPI_Comm intra_MPI_comm
MPI intra-communicator that spans all ranks associated with this code.
Definition: mdi_global.h:222
Definition: mdi_global.h:111
int(* mpi4py_send_callback)(void *, int, int, int, MDI_Comm_Type)
Python callback pointer for MPI_Send.
Definition: mdi_global.h:206
int(* language_on_destroy)(int)
Function pointer to the language-specific destructor function.
Definition: mdi_global.h:202
char *** plugin_argv_ptr
Argument vector for plugin command-line options.
Definition: mdi_global.h:188
int id
ID of this method.
Definition: mdi_global.h:127
int name_length
The value of MDI_NAME_LENGTH for the connected code.
Definition: mdi_global.h:156
int vector_delete(vector *v, int index)
Remove an element from a vector.
Definition: mdi_global.c:93
int mdi_debug(const char *message,...)
Print a debug message.
Definition: mdi_global.c:820
size_t current_key
If the array has a currently active component, this is that value.
Definition: mdi_global.h:97
int get_code(size_t code_id, code **ret_code)
Get a code from a code handle Returns a pointer to the code.
Definition: mdi_global.c:359
int language
Native language of this code.
Definition: mdi_global.h:241
int debug_mode
Flag for whether this code is running in debug mode.
Definition: mdi_global.h:261
int command_length
The value of MDI_COMMAND_LENGTH for the connected code.
Definition: mdi_global.h:158
int ipi_compatibility
Flag for whether MDI is running in i-PI compatibility mode.
Definition: mdi_global.h:249
int get_communicator(size_t code_id, MDI_Comm_Type comm_id, communicator **comm_ptr)
Get a communicator from a communicator handle Returns a pointer to the communicator.
Definition: mdi_global.c:660
int world_rank
Rank of this process within MPI_COMM_WORLD.
Definition: mdi_global.h:233
int get_callback_index(node *n, const char *callback_name, int *callback_index)
Determine the index of a callback within a node.
Definition: mdi_global.c:216
size_t id
ID of this element.
Definition: mdi_global.h:115
int get_current_code(code **this_code_ptr)
Get the currently active code Returns a pointer to the code.
Definition: mdi_global.c:387
int tcp_initialized
Flag whether this code has previously initialized TCP.
Definition: mdi_global.h:253
int new_code(size_t *code_id)
Create a new code structure and add it to the list of codes Returns the index of the new code.
Definition: mdi_global.c:267
int get_command_index(node *n, const char *command_name, int *command_index)
Determine the index of a command within a node.
Definition: mdi_global.c:189
size_t stride
Size of each element.
Definition: mdi_global.h:91
unsigned char * data
The elements stored by this vector.
Definition: mdi_global.h:89
MDI_Comm_Type id
MDI_Comm handle that corresponds to this communicator.
Definition: mdi_global.h:152
int is_accepted
Indicate whether this communicator has been accepted yet.
Definition: mdi_global.h:154
int delete_communicator(size_t code_id, MDI_Comm_Type comm_id)
Delete a communicator.
Definition: mdi_global.c:694
char * hostname
Hostname of the driver.
Definition: mdi_global.h:194
size_t size
Number of elements actually stored.
Definition: mdi_global.h:95
size_t id
Handle for this code.
Definition: mdi_global.h:235
int(* driver_callback_f90)(void *)
Function pointer to the actual callback for Fortran drivers.
Definition: mdi_global.h:196
int vector_push_back(vector *v, void *element)
Append a new element to the end of the vector.
Definition: mdi_global.c:60
char name[MDI_COMMAND_LENGTH_]
Name of the node.
Definition: mdi_global.h:163
int delete_method(size_t code_id, int method_id)
Delete a method Returns 0 on success.
Definition: mdi_global.c:540
void * execute_command_obj
Pointer to the class object that is passed to any call to execute_command.
Definition: mdi_global.h:176
int intra_rank
Rank of this process within its associated code.
Definition: mdi_global.h:243
int(* on_selection)()
Function pointer for method initialization work.
Definition: mdi_global.h:121
int new_method(size_t code_id, int method_id, int *id_ptr)
Create a new method structure and add it to the vector of methods Returns the handle of the new metho...
Definition: mdi_global.c:482
size_t code_id
Handle for the id of the associated code.
Definition: mdi_global.h:146
void * data
The actual data for this element.
Definition: mdi_global.h:113
int get_method(size_t code_id, int method_id, method **method_ptr)
Get a method from a method handle Returns a pointer to the method.
Definition: mdi_global.c:507
vector * nodes
The nodes supported by the connected code.
Definition: mdi_global.h:136
int is_library
Flag whether this code is being used as a library 0: Not a library 1: Is an ENGINE library,...
Definition: mdi_global.h:229
int(* mpi4py_gather_names_callback)(void *, void *, int *, int *)
Python callback pointer for gathering names.
Definition: mdi_global.h:210
void mdi_warning(const char *message)
Print warning message.
Definition: mdi_global.c:810
int next_comm
The handle of the next communicator.
Definition: mdi_global.h:239
int convert_buf_datatype(void *recvbuf_in, MDI_Datatype_Type recvtype, void *sendbuf_in, MDI_Datatype_Type sendtype, int count)
Convert a buffer from one datatype to another.
Definition: mdi_global.c:964
Definition: mdi_global.h:170
vector * methods
Vector containing all supported methods.
Definition: mdi_global.h:182
int world_size
Size of MPI_COMM_WORLD.
Definition: mdi_global.h:231
int vector_init(vector *v, size_t stride)
Initialize memory allocation for a vector structure.
Definition: mdi_global.c:34
int datatype_info(MDI_Datatype_Type datatype, size_t *size, MDI_Datatype_Type *base)
Get information about an MDI_Datatype.
Definition: mdi_global.c:847
int port
Port over which the driver will listen.
Definition: mdi_global.h:259
int(* execute_command_wrapper)(const char *, MDI_Comm_Type, void *)
Function pointer to the language-specific wrapper for the execute_command function.
Definition: mdi_global.h:198
int(* mpi4py_recv_callback)(void *, int, int, int, MDI_Comm_Type)
Python callback pointer for MPI_Recv.
Definition: mdi_global.h:204
int method_id
Communication method used by this communicator.
Definition: mdi_global.h:150
sock_t tcp_socket
Socket for TCP connections.
Definition: mdi_global.h:224
int free_methods_vector(vector *v)
Free the memory associated with a methods vector.
Definition: mdi_global.c:595
int delete_code(size_t code_id)
Delete a code Returns 0 on success.
Definition: mdi_global.c:401
Definition: mdi_global.h:87
MDI_execute_command_type execute_command
Function pointer to the generic execute_command_function.
Definition: mdi_global.h:200
int called_set_execute_command_func
Flag whether this code has called set_execute_command_func.
Definition: mdi_global.h:245
vector * nodes
Vector containing all nodes supported by this code.
Definition: mdi_global.h:178
vector codes
Vector containing all codes that have been initiailized on this rank. Typically, this will only inclu...
Definition: mdi_global.c:22
int method_id
Communication method.
Definition: mdi_global.h:129
char * plugin_path
Path to the plugins available to this code.
Definition: mdi_global.h:184
int selected_method_id
ID of the method being used for inter-code communication.
Definition: mdi_global.h:247
int(* py_launch_plugin_callback)(void *, void *, void *, int)
Python callback pointer for MPI_Comm_barrier.
Definition: mdi_global.h:220
Definition: mdi_global.h:119
char ** plugin_unedited_options_ptr
Unedited command-line options for currently running plugin.
Definition: mdi_global.h:190
int initialized_mpi
Flag for whether MDI called MPI_Init.
Definition: mdi_global.h:251
int(* mpi4py_rank_callback)(int)
Python callback pointer for MPI_Comm_rank.
Definition: mdi_global.h:214
Definition: mdi_global.h:161
int datatype_mpitype(MDI_Datatype_Type datatype, MPI_Datatype *mpitype)
Get the MPI datatype that corresponds to an MDI datatype.
Definition: mdi_global.c:915
vector * callbacks
Vector containing all the callbacks associated with this node.
Definition: mdi_global.h:167
int(* mpi4py_barrier_callback)(int)
Python callback pointer for MPI_Comm_barrier.
Definition: mdi_global.h:218
int test_initialized
Flag whether this code has previously initialized TEST.
Definition: mdi_global.h:257
int mdi_version[3]
The MDI version of the connected code.
Definition: mdi_global.h:134